Add code into the head of the page:
<style>
.message {
font-size: 1.2em;
margin-top: 10px;
color: green;
}
.error {
color: red;
}
</style>
<script>
function validateText() {
const userInput = document.getElementById("userText").value;
const correctText = "correct text"; // Replace with the actual correct text
const messageElement = document.getElementById("message");
if (userInput === correctText) {
messageElement.textContent = "Text is correct!";
messageElement.classList.remove("error");
} else {
messageElement.textContent = "Text is incorrect. Please try again.";
messageElement.classList.add("error");
}
}
</script>
Where it says “correct text”, replace with the answer you want. This where you also edit the style of it.
Then in the body where you want to input to occur. Add the “Code Embed” module under the “Advanced” section.
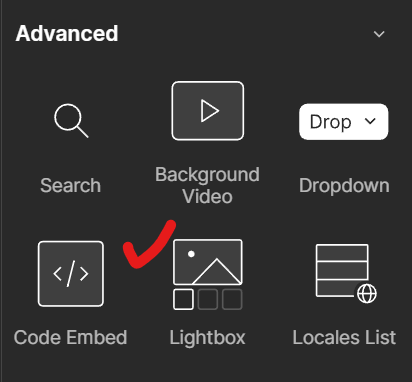
Then add the following code:
<form onsubmit="event.preventDefault(); validateText();">
<label for="userText">Enter text:</label>
<input type="text" id="userText" name="userText" required>
<button type="submit">Validate</button>
</form>
<div id="message" class="message"></div>
This is how it should look like.

You can test it out and check it works. Hope that helps! Happy creating.
Leave a Reply
You must be logged in to post a comment.